Whether you are interested in buying, or selling, property via Rightmove, you might want to download the images. Now sure, you can do this one-by-one, by right clicking on each photo (when viewed full screen) and choose to copy/save the photos, but that’s a very manual task, which will take you time.
This type of manual work is fine as a one off, but to do it regulary is very time consuming and boring.
The good news is, I’ve made it easy for you by writing the below PowerShell script. I’ll talk you through what it does, but if you just want the end result, skip to the end of this article.
This article has been updated to replace Invoke-WebRequest with Start-BitsTransfer, since some readers reported not having IE installed or not actioning the first run steps within IE, which prevented the script from running.
PowerShell ask for a URL
So the script needs to know what the URL to the rightmove page is, so to do this, we just ask for user input using the Read-Host cmdlet, and store it in a variable called $url, as shown below:
$url=Read-Host -Prompt 'Input a Right Move URL and press enter'
You’ll then see the below, where you can paste your URL in, and press enter:

Download the HTML source code of the website
To download the HTML source code of the listing, we’ll use Start-BitsTransfer, but first, we need to set the TLS protocols that we want to use. You may not need this, but depending on your version of PowerShell, you might, so lets include it:
[Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Tls -bor [Net.SecurityProtocolType]::Tls11 -bor [Net.SecurityProtocolType]::Tls12
Then, let’s download the data via an Start-BitsTransfer, and save it to a variable called $html
$webreq = Start-BitsTransfer $url
$html=$webreq.Content
Folder structure
Now we have the HTML source code, let’s think about how to organise the downloaded photo, and for this I believe it’s best to create sub folders. For this example, I’ll use the title of the listing, which is withing the <title></title> tags of the pages HTML.
There are various ways to get the content between two strings, or HTML tags in this case, but I like to do it via a function. For this example, we’ll pass in the opening tag/string (<title>), closing tag/string (</title>) and the html source code.
Function:
function GetStringBetweenTwoStrings($openingtag, $closingtag, $html){
#Regex pattern to compare two strings
$pattern = "$openingtag(.*?)$closingtag"
#Perform the opperation
$result = [regex]::Match($html,$pattern).Groups[1].Value
#Return result
return $result
}
Getting the title from the HTML source code:
$title=GetStringBetweenTwoStrings -openingtag "<title>" -closingtag "</title>" -html $html
We’ll take the new $title variable, and prepend a date string to it, just in case you choose to re-download the same listing again:
$foldername="$((Get-Date).ToString('yyyyMMdd'))_$($title -replace " ","_")"
To create the folder, first we need to check if it’s already there, although this is unlikely since we have a date string, but it is possible, and if not, create it:
$saveto=".\RIGHTMOVE\$($foldername)"
[System.IO.Directory]::Exists($saveto)
if (!(Test-Path $saveto)) {
Write-Warning "$saveto does not exist, it will be created now"
New-Item -Path $saveto -ItemType Directory
}
Finding the images from the HTML code
To find all of the images in the HTML source code of the Rightmove listing, we need to find all of the meta tags with the og:image property. The easiest way to do this is with regex, and we’ll filter those into a different variable called $metatags
$metatags = ([regex]'<meta property="og:image" ((.|\n|\r)+?)\/>').Matches($html)
Saving the images with PowerShell
The Rightmove photos wont necessarily be named in a friendly way, so as we save them, we’ll append a numeric value, so they are in order.
We’ll start by looping through each of the already filtered og:image meta tags using ForEach, and for each individual item, we’ll strip out what we don’t need to get the URL and lastly download the image. We could have used the function to return the URL, but I’ve done this way to show you another approach, even though it’s not normally what I would do, but it does work:
[int]$int=1
ForEach ($metatag in $metatags)
{
$imageurl=(($metatag -replace "`"/>","") -replace "<meta property=`"og:image`" content=`"","")
Write-Host $imageurl
Start-BitsTransfer $imageurl -Destination "$($saveto)`\Image_$('{0:d2}' -f [int]$int).jpg" -MaxDownloadTime 1500
$int++
}
The full PowerShell script for downloading Rightmove listing photos
Save this text as in a text file, then make sure you rename it so the extension is .ps1 instead of .txt
CLEAR
$url=Read-Host -Prompt 'Input a Right Move URL and press enter'
function GetStringBetweenTwoStrings($openingtag, $closingtag, $html)
{
$pattern = "$openingtag(.*?)$closingtag"
$result = [regex]::Match($html,$pattern).Groups[1].Value
return $result
}
[Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Tls -bor [Net.SecurityProtocolType]::Tls11 -bor [Net.SecurityProtocolType]::Tls12
$webreq = Start-BitsTransfer $url
$html=$webreq.Content #| Out-File C:\temp\output.txt
$title=GetStringBetweenTwoStrings -openingtag "<title>" -closingtag "</title>" -html $html
$foldername="$((Get-Date).ToString('yyyyMMdd'))_$($title -replace " ","_")"
Write-Host $foldername
$saveto=".\RIGHTMOVE\$($foldername)"
[System.IO.Directory]::Exists($saveto)
if (!(Test-Path $saveto)) {
Write-Warning "$saveto does not exist, it will be created now"
New-Item -Path $saveto -ItemType Directory
}
Write-Host "Title: $title"
Write-Host "URL: $url"
$metatags = ([regex]'<meta property="og:image" ((.|\n|\r)+?)\/>').Matches($html)
[int]$int=1
ForEach ($metatag in $metatags)
{
$imageurl=(($metatag -replace "`"/>","") -replace "<meta property=`"og:image`" content=`"","")
Write-Host $imageurl
Start-BitsTransfer $imageurl -Destination "$($saveto)`\Image_$('{0:d2}' -f [int]$int).jpg" -MaxDownloadTime 1500
$int++
}
When run, you will see an output similar to this:
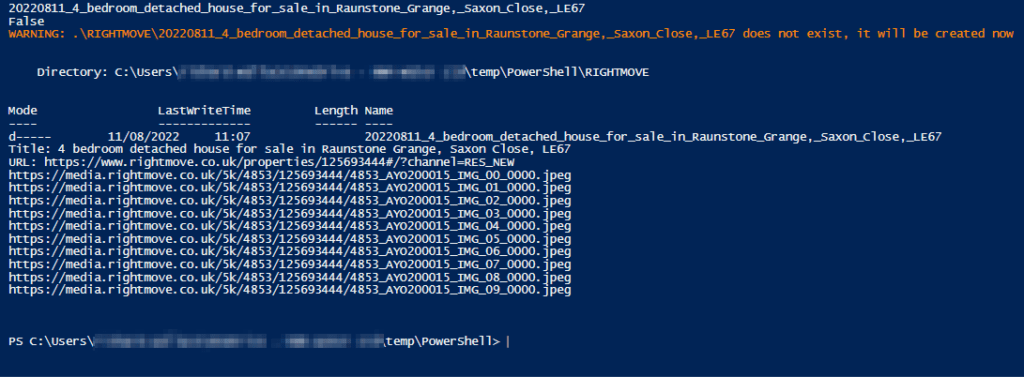
The photos will be saved in a photo like this:
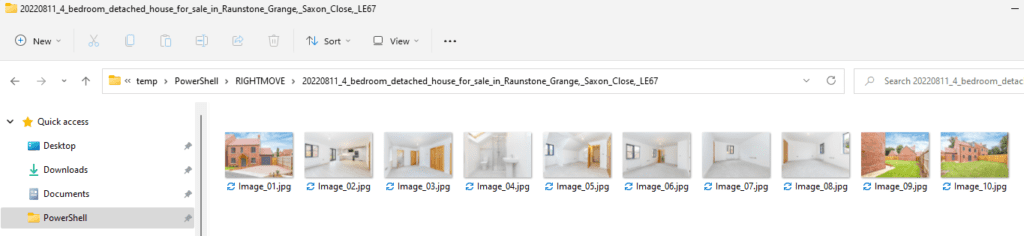
If that doesn’t work for you, try the old Invoke-WebRequest approach:
CLEAR
$url=Read-Host -Prompt 'Input a Right Move URL and press enter'
function GetStringBetweenTwoStrings($openingtag, $closingtag, $html)
{
$pattern = "$openingtag(.*?)$closingtag"
$result = [regex]::Match($html,$pattern).Groups[1].Value
return $result
}
[Net.ServicePointManager]::SecurityProtocol = [Net.SecurityProtocolType]::Tls -bor [Net.SecurityProtocolType]::Tls11 -bor [Net.SecurityProtocolType]::Tls12
$webreq = Invoke-WebRequest $url
$html=$webreq.Content #| Out-File C:\temp\output.txt
$title=GetStringBetweenTwoStrings -openingtag "<title>" -closingtag "</title>" -html $html
$foldername="$((Get-Date).ToString('yyyyMMdd'))_$($title -replace " ","_")"
Write-Host $foldername
$saveto=".\RIGHTMOVE\$($foldername)"
[System.IO.Directory]::Exists($saveto)
if (!(Test-Path $saveto)) {
Write-Warning "$saveto does not exist, it will be created now"
New-Item -Path $saveto -ItemType Directory
}
Write-Host "Title: $title"
Write-Host "URL: $url"
$metatags = ([regex]'<meta property="og:image" ((.|\n|\r)+?)\/>').Matches($html)
[int]$int=1
ForEach ($metatag in $metatags)
{
$imageurl=(($metatag -replace "`"/>","") -replace "<meta property=`"og:image`" content=`"","")
Write-Host $imageurl
Invoke-WebRequest -Uri $imageurl -OutFile "$($saveto)`\Image_$('{0:d2}' -f [int]$int).jpg" -TimeoutSec 1500
$int++
}
rightmove_image_downloader(this link opens in a new window)byrswio(this link opens in a new window)
A PowerShell script that allows you to download and organise images from Rightmove listings using just the URL
Sounds such a great tool – but I get this error message – any advice how to overcome this?
Invoke-WebRequest : The response content cannot be parsed because the Internet Explorer engine is not available, or
Internet Explorer’s first-launch configuration is not complete. Specify the UseBasicParsing parameter and try again.
At line:13 char:11
+ $webreq = Invoke-WebRequest $url
+ ~~~~~~~~~~~~~~~~~~~~~~
+ CategoryInfo : NotImplemented: (:) [Invoke-WebRequest], NotSupportedException
+ FullyQualifiedErrorId : WebCmdletIEDomNotSupportedException,Microsoft.PowerShell.Commands.InvokeWebRequestComman
d
You could try changing Invoke-WebRequest to Start-BitsTransfer, or open Internet Explorer and deal with the first run prompts. Either should work fine, and in fact I may update the article to use that instead
I’ve updated the article now, so it uses Start-BitsTransfer instead of Invoke-WebRequest.
Hi
I am getting the following error
Input a Right Move URL and press enter: https://www.rightmove.co.uk/properties/118798721#
Start-BitsTransfer : HTTP status 404: The requested URL does not exist on the server.
At C:\Users\Georges\Documents\powerscript.ps1:13 char:11 + $webreq = Start-BitsTransfer $url + ~~~~~~~~~~~~~~~~~~~~~~~ + CategoryInfo : InvalidOperation: (:) [Start-BitsTransfer], Exception + FullyQualifiedErrorId : StartBitsTransferCOMException,Microsoft.BackgroundIntelligentTransfer.Management.NewBits TransferCommand 20230122_
True
Title:
URL: https://www.rightmove.co.uk/properties/118798721#
Strange that you see this, as it works for me. The error 404 message implies that the page cannot be found. Perhaps make sure there’s not space at the start, although I wouldn’t expect it to be that.
You can also try the old Invoke-WebRequest approach, which I’ve just added to the bottom of the article for you.